API Documentation Classic and REST Versions
Email Payments Intro
The L2P Email Payment Web Service is a set of methods used to submit debit and credit payment requests.
Thru this feature rich tool you can take advantage of things like recurring payments, convenience fees, virtual attachments, password protection of those attachments and much more.
To get started follow these instructions:
- Review this documentation in its entirety
-
Download the L2P Payment Webservice Sample Application by clicking here
To obtain the corresponding WSDL for testing purposes click here
- Download the WSDL file
- Once you are ready to move to production, please register your client(s) by clicking “Register” on www.linked2pay.com and completing our simple 5 step registration process.
- For more information about linked2pay's API please email us at: supportteam@linked2pay.com
If you would like to try out the JSON-oriented REST Web Service Features, click here to try, no account registration needed.
Methods
Name | Description |
---|---|
submitCreditRequest |
Submit credit request to credit contact account and debit client (merchant) account. This call sends email to contact. Email text includes link to the L2P payment page. On receiving email contact goes to the payment page, types in his account, authorizes the transaction and system initiates ACH credit transaction to this account. If contact does not accept ACH transaction within seven days then a check for the requested amount will be sent to contact by mail to the address specified in this credit request. |
submitDebitRequest |
Submit debit request to debit contact account and credit client (merchant) account. This call sends email to contact. Email subject and content are explicitly specified as web service parameters. Email text includes link to the L2P payment page. On receiving email contact goes to the payment page, types in his account or credit card information, authorizes the transaction and system initiates ACH or credit card transaction. |
submitDebitRequestByTemplate |
Submit debit request to debit contact account and credit client (merchant) account. This call sends email to contact. Email is constructed based on template previously defined by the client and specified as web service parameter. Email text includes link to the L2P payment page. On receiving email contact goes to the payment page, types in his account or credit card information, authorizes the transaction and system initiates ACH or credit card transaction. |
submitRequestAttachment | Upload document (say invoice) attached to a particular L2P Request. Customer will be able to open and view attached document through the L2P payment page. |
The ‘Submit Credit Request' method can be used to make a payment to your Contact(s). This is accomplished by initiating a debit to your bank account and a corresponding credit to your Contact(s) bank account.
This call sends an email to your Contact(s). Included in the Email Request is a link to the linked2pay payment page. Upon receipt of the email request the Contact(s) can click the payment link and view the accept payment page. Here the Contact(s) will, 1) specify the Route and Account which they want to accept payment to, and, 2) authorize/accept the ACH credit transaction to the specified account.
If a Contact does not accept the ACH transaction within seven days then a check in the amount of your request will be mailed to the address you provided for this Contact.
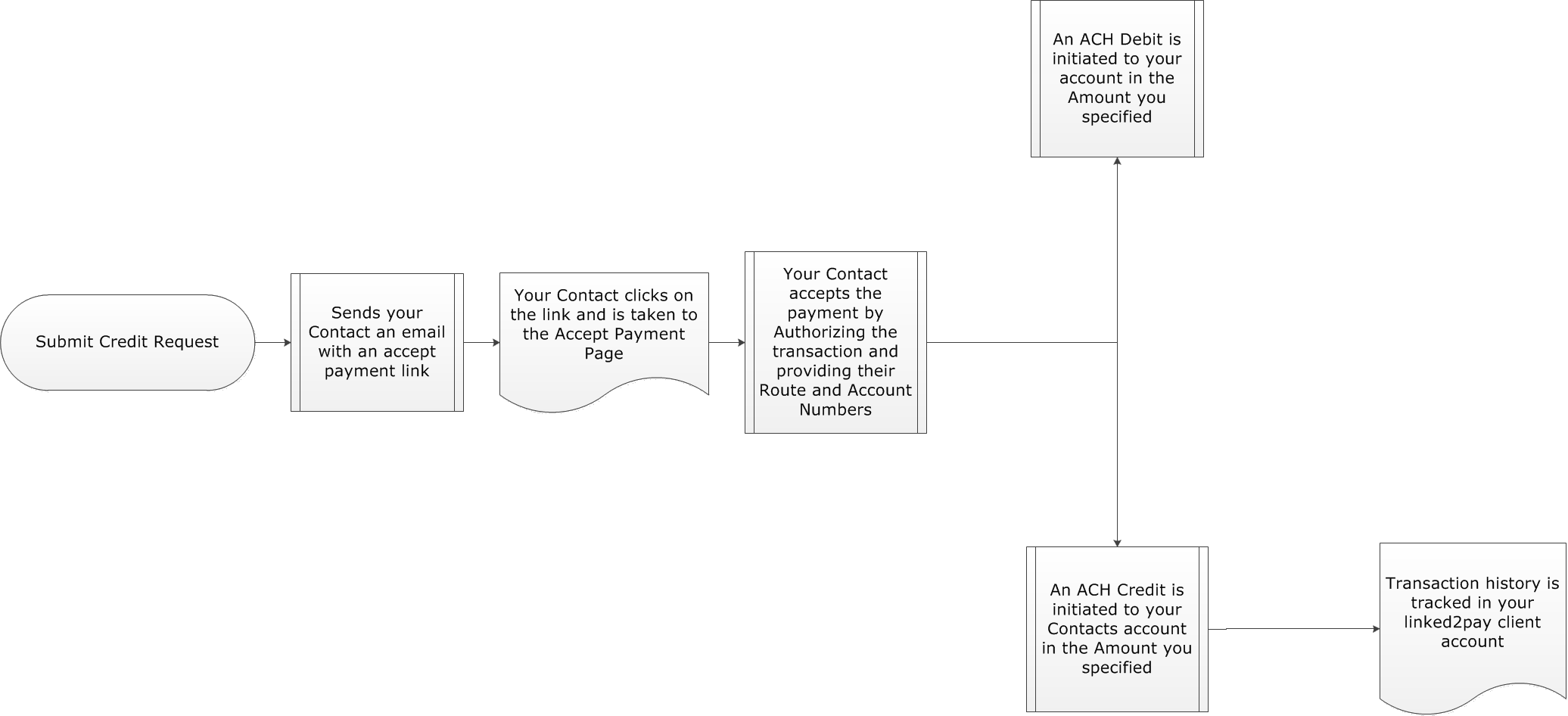
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
request | L2PCreditRequest | Input | Data objects that includes credit request attributes. |
Returns
A submitCreditRequestReturn element has the structure defined by the following table:
Name | Type | Description |
---|---|---|
errorCode | int |
Negative error code or 0 if call is successful. See error codes list in the attachment. |
errorMsg | string | Short explanation of the error code. |
l2pContactId | long | Unique contact identifier. |
l2pRequestId | long | Unique payment request identifier. |
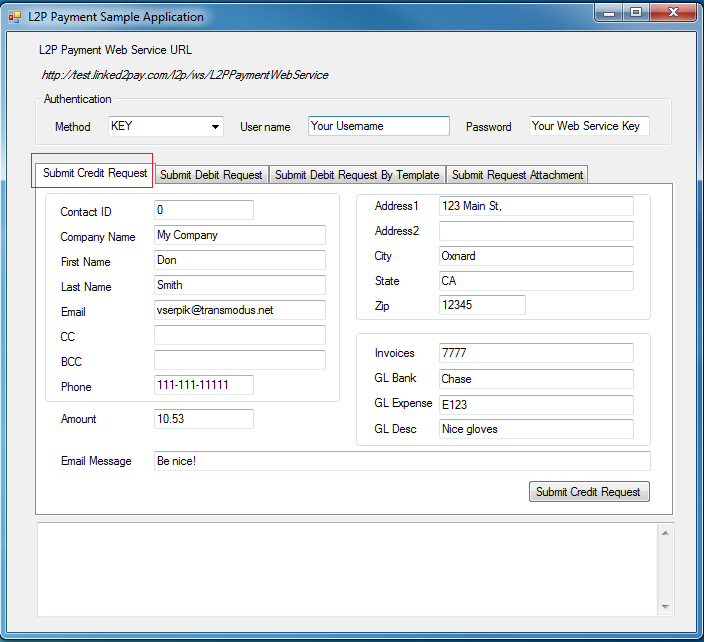
Below is the code sample in PHP
<?php define('L2P_SERVICE_PATH', 'http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT', L2P_SERVICE_PATH.'services/L2PPaymentWebService'); define('L2P_WSDL', L2P_SERVICE_PATH.'wsdl/L2PPaymentWebService.wsdl'); // SOAP client to be used on pages $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object to be used on pages $auth = new StdClass(); $auth->login = "mikel1"; $auth->AuthenticationMethod = "KEY"; $auth->password = "0123456789ABCDEF"; $l2pCreditRequest = new StdClass(); $l2pCreditRequest->contactId = 0; $l2pCreditRequest->companyName = 'My Company'; $l2pCreditRequest->firstName = 'Don'; $l2pCreditRequest->lastName = 'Smith'; $l2pCreditRequest->email = 'vserpik@transmodus.net'; $l2pCreditRequest->cc = ''; $l2pCreditRequest->bcc = ''; $l2pCreditRequest->phone = '111-111-11111'; $l2pCreditRequest->amount = 1234.12; $l2pCreditRequest->invoices = '7777'; $l2pCreditRequest->address1 = '123 Main St.'; $l2pCreditRequest->address2 = ''; $l2pCreditRequest->city = 'Oxnard'; $l2pCreditRequest->state = 'CA'; $l2pCreditRequest->zip = '12345'; $l2pCreditRequest->glBank = 'Chase'; $l2pCreditRequest->glExpense = 'E123'; $l2pCreditRequest->glDesc = 'Nice gloves'; $l2pCreditRequest->emailMessage = 'Be nice'; $params = new StdClass(); $params->auth = $auth; $params->request = $l2pCreditRequest; $result = $client->submitCreditRequest($params); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Credit Request</title> </head> <body> <div> <p>Response:</p> <div> <?php print_results($result->submitCreditRequestReturn); ?> </div> </div> </body> </html> <?php // function to print results function print_results($obj) { foreach ($obj as $k => $v) { print $k.' -- <b>'.$v.'</b><br />'."\n"; } } ?>
Below is the sample code in C#
// Web Reference has been added as: // reference name: L2PReportPaymentWebService // URL: http://test.transmodus.net/jcx/soa/test/wsdl/L2PPaymentWebService.wsdl // More on adding service references see: // http://msdn.microsoft.com/en-us/library/bb628649.aspx // http://msdn.microsoft.com/en-us/library/bb628652.aspx using System; using L2PPaymentSampleApplication.L2PPaymentWebService; namespace L2PPaymentSampleApplication { class Program { static void Main(string[] args) { L2PPaymentWebServiceService l2pPaymentWebService = new L2PPaymentWebServiceService(); ; l2pPaymentWebService.Url = "http://www.linked2pay.com/l2p/ws/L2PPaymentWebService"; L2PPaymentWebService.L2PAuthenticationData auth = new L2PPaymentWebService.L2PAuthenticationData(); L2PPaymentWebService.L2PCreditRequest l2pCreditRequest = new L2PPaymentWebService.L2PCreditRequest(); auth.login = "mikel1"; // auth.AuthenticationMethod = "KEY"; // password = "0123456789ABCDEF"; L2PReportWebService.fingerprintBlock fingerprintBlock = new L2PReportWebService.fingerprintBlock(); FingerprintGenerator fingerprintGenerator = new FingerprintGenerator(); fingerprintBlock.sequence = fingerprintGenerator.GetSuquenceNumber(); fingerprintBlock.fingerprint = fingerprintGenerator.GenerateFingerPrint( int)fingerprintBlock.sequence, fingerprintBlock.timesatmp.ToString(), "0123456789ABCDEF", auth.login); auth.fingerprint = fingerprintBlock; l2pCreditRequest.contactId = 0; l2pCreditRequest.companyName = "My Company"; l2pCreditRequest.firstName = "Don"; l2pCreditRequest.lastName = "Smith"; l2pCreditRequest.email = "vserpik@transmodus.net";; l2pCreditRequest.cc = ""; l2pCreditRequest.bcc = ""; l2pCreditRequest.phone = "111-111-11111"; l2pCreditRequest.amount = 1234.12; l2pCreditRequest.invoices = "7777"; l2pCreditRequest.address1 = "123 Main St."; l2pCreditRequest.address2 = ""; l2pCreditRequest.city = "Oxnard"; l2pCreditRequest.state = "CA"; l2pCreditRequest.zip = "12345"; l2pCreditRequest.glBank = "Chase"; l2pCreditRequest.glExpense = "E123"; l2pCreditRequest.glDesc = "Nice gloves"; l2pCreditRequest.emailMessage = "Be nice"; L2PPaymentWebService.L2PCreditResponse l2pCreditResponse = l2pPaymentWebService.submitCreditRequest(auth, l2pCreditRequest); Console.WriteLine("Contact ID: " + l2pCreditResponse.l2pContactId); Console.WriteLine("Request ID: " + l2pCreditResponse.l2pRequestId); } } }
The 'Submit Debit Request' method can be used to submit a request to debit your Contact(s) account and credit your bank/merchant account. This call will send an email to your Contact(s). The email Subject and Content are explicitly specified as web service parameters.
Included in the email content is a link to the linked2pay payment page. Upon receipt of the email request your Contact(s) can follow the link to the payment page where they will, 1) provide the route and account number or credit card they want to debit or charge, and 2) authorize the transaction against their account. linked2pay will then initiate an ACH or credit card transaction in the amount of your request.
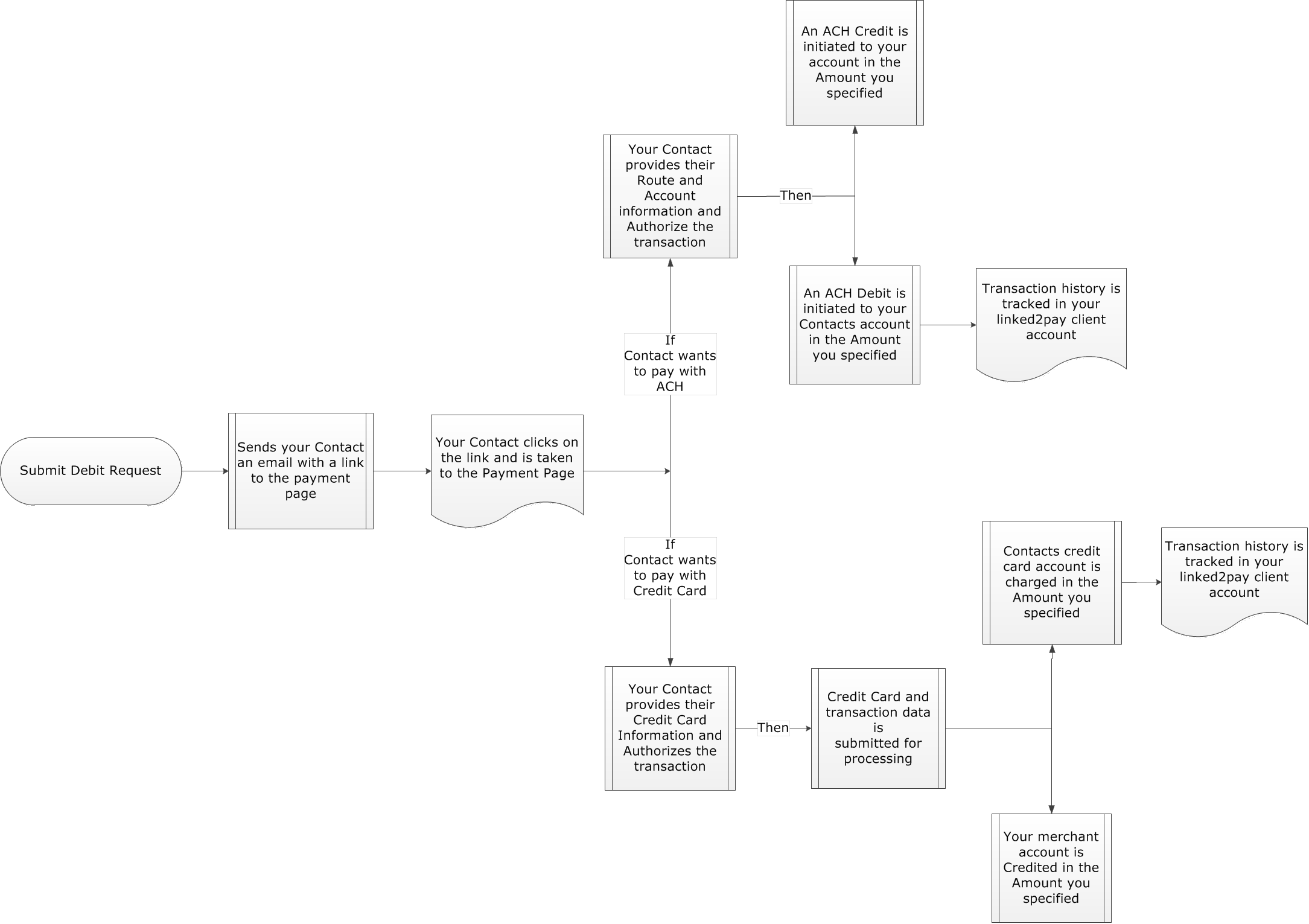
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
request | L2PDebitRequest | Input | Data objects that includes debit request attributes. |
Returns
A submitDebitRequestReturn element has the structure defined by the following table:
Name | Type | Description |
---|---|---|
errorCode | int |
Negative error code or 0 if call is successful. See error codes list in the attachment. |
errorMsg | string | Short explanation of the error code. |
l2pContactId | long | Unique contact identifier. |
l2pRequestId | long | Unique payment request identifier. |
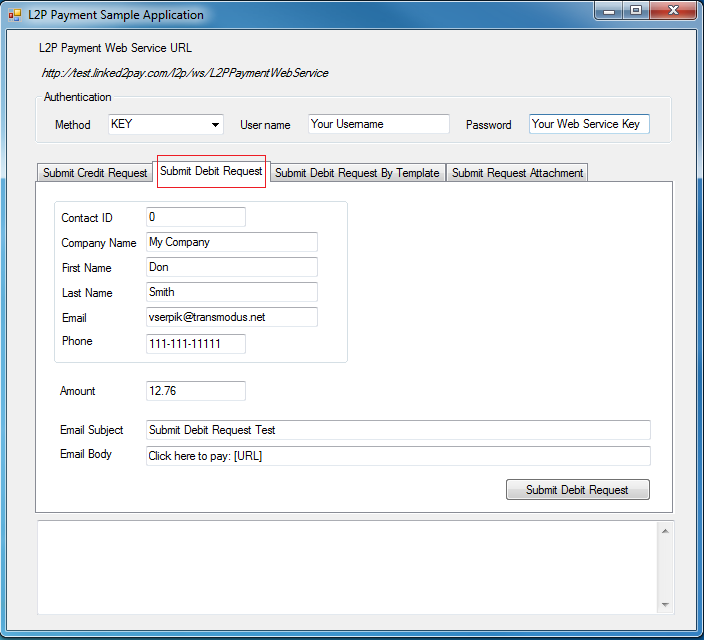
Below is the code sample in PHP
<?php define('L2P_SERVICE_PATH', 'http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT', L2P_SERVICE_PATH.'services/L2PPaymentWebService'); define('L2P_WSDL', L2P_SERVICE_PATH.'wsdl/L2PPaymentWebService.wsdl'); // SOAP client to be used on pages $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object to be used on pages $auth = new StdClass(); $auth->login = "mikel1"; $auth->AuthenticationMethod = "KEY"; $auth->password = "0123456789ABCDEF"; $l2pDebitRequest = new StdClass(); $l2pDebitRequest->contactId = 0; $l2pDebitRequest->companyName = 'My Company'; $l2pDebitRequest->firstName = 'Don'; $l2pDebitRequest->lastName = 'Smith'; $l2pDebitRequest->email = 'vserpik@transmodus.net'; $l2pDebitRequest->phone = '111-111-11111'; $l2pDebitRequest->amount = 10.50; $l2pDebitRequest->emailSubject = 'PHP Submit Debit Request Test'; $l2pDebitRequest->emailBody = 'Click here to pay: [URL]'; $params = new StdClass(); $params->auth = $auth; $params->request = $l2pDebitRequest; $result = $client->submitDebitRequest($params); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Credit Request</title> </head> <body> <div> <p>Response:</p> <div> <?php print_results($result->submitDebitRequestReturn); ?> </div> </div> </body> </html> <?php // function to print results function print_results($obj) { foreach ($obj as $k => $v) { print $k.' -- <b>'.$v.'</b><br />'."\n"; } } ?>
Below is the sample code in C#
// Web Reference has been added as: // reference name: L2PReportPaymentWebService // URL: http://test.transmodus.net/jcx/soa/test/wsdl/L2PPaymentWebService.wsdl // More on adding service references see: // http://msdn.microsoft.com/en-us/library/bb628649.aspx // http://msdn.microsoft.com/en-us/library/bb628652.aspx using System; using L2PPaymentSampleApplication.L2PPaymentWebService; namespace L2PPaymentSampleApplication { class Program { static void Main(string[] args) { L2PPaymentWebServiceService l2pPaymentWebService = new L2PPaymentWebServiceService(); ; L2PPaymentWebService.L2PAuthenticationData auth = new L2PPaymentWebService.L2PAuthenticationData(); l2pPaymentWebService.Url = "http://www.linked2pay.com/l2p/ws/L2PPaymentWebService"; L2PPaymentWebServiceService l2pPaymentWebService = new L2PPaymentWebServiceService(); auth.login = "mikel1"; // auth.AuthenticationMethod = "KEY"; // password = "0123456789ABCDEF"; L2PReportWebService.fingerprintBlock fingerprintBlock = new L2PReportWebService.fingerprintBlock(); FingerprintGenerator fingerprintGenerator = new FingerprintGenerator(); fingerprintBlock.sequence = fingerprintGenerator.GetSuquenceNumber(); fingerprintBlock.fingerprint = fingerprintGenerator.GenerateFingerPrint( (int)fingerprintBlock.sequence, fingerprintBlock.timesatmp.ToString(), "0123456789ABCDEF", auth.login); auth.fingerprint = fingerprintBlock; l2pDebitRequest.contactId = 0; l2pDebitRequest.companyName = "My Company"; l2pDebitRequest.firstName = "Don"; l2pDebitRequest.lastName = "Smith"; l2pDebitRequest.email = "vserpik@transmodus.net";; l2pDebitRequest.phone = "111-111-11111"; l2pDebitRequest.amount = 10.50; l2pDebitRequest.emailSubject = "PHP Submit Debit Request Test"; l2pDebitRequest.emailBody = "Click here to pay: [URL]"; L2PPaymentWebService.L2PDebitResponse l2pDebitResponse = l2pPaymentWebService.submitDebitRequest(auth, l2pDebitRequest); Console.WriteLine("Error code: " + l2pDebitResponse.errorCode); Console.WriteLine("Contact ID: " + l2pDebitResponse.l2pContactId); Console.WriteLine("Request ID: " + l2pDebitResponse.l2pRequestId); } } }
The ‘Submit Debit Request By Template’ method can be used to send a specific email template from your linked2pay client site to request a payment from your Contact(s). Once a payment is submitted by the Contact(s) a transaction will be initiated to Debit your Contact(s) account and Credit your bank/merchant account.
This call will send your Contact(s) an email request based on the Email Template you specify in the web service parameters. The body of the email will include a link to the linked2pay payment page. Upon receipt of the email request, your Contact(s) will click on the payment page link and 1) provide the bank route and account number or credit card they want to debit or charge, and, 2) authorize the transaction. linked2pay will then initiate an ACH or credit card transaction in the amount of your request.
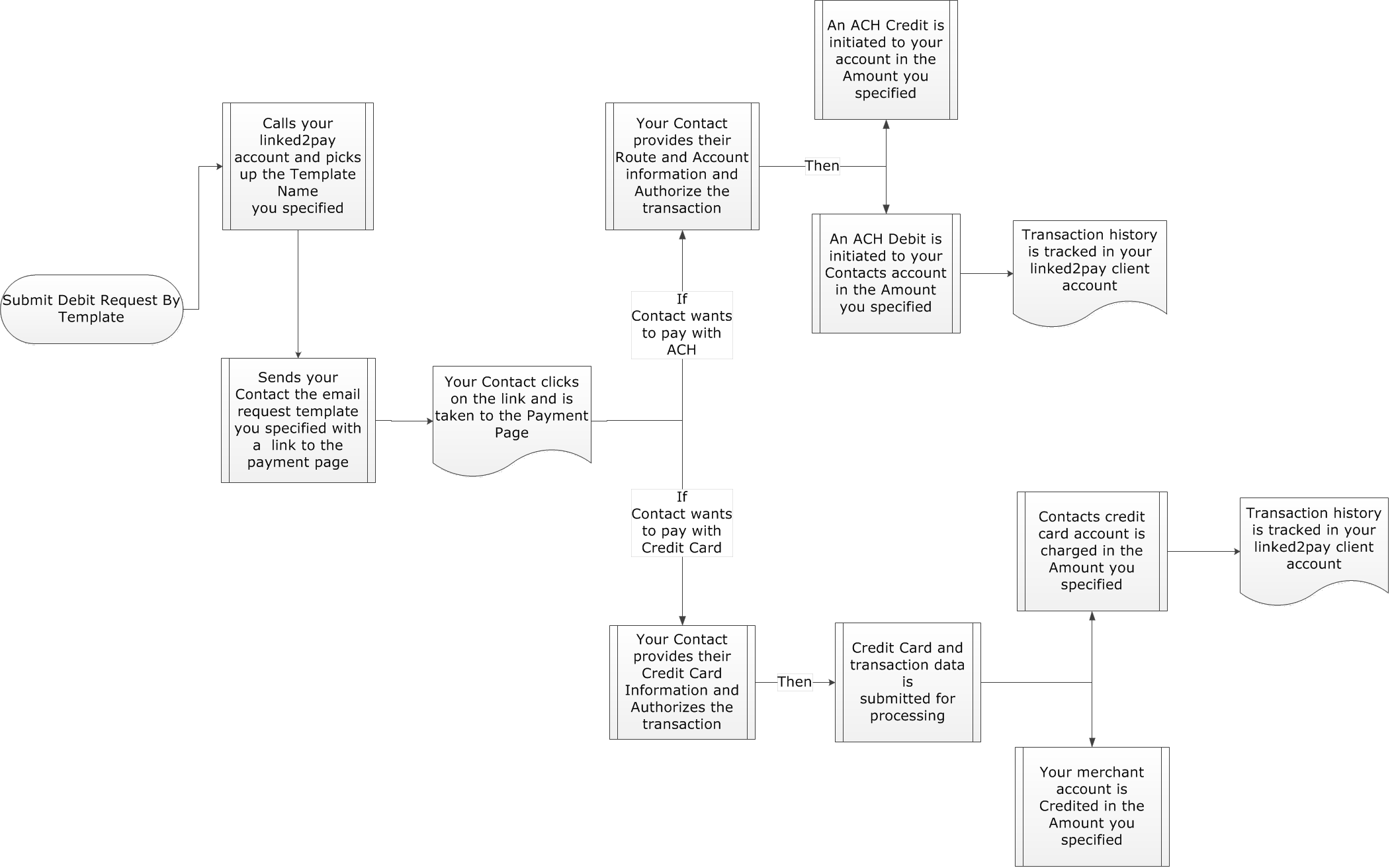
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
request | L2PDebitRequestByTemplate | Input | Data objects that includes debit request attributes. |
Returns
A submitDebitRequestByTemplateReturn element has the structure defined by the following table.
Name | Type | Description |
---|---|---|
errorCode | int |
Negative error code or 0 if call is successful. See error codes list in the attachment. |
errorMsg | string | Short explanation of the error code. |
l2pContactId | long | Unique contact identifier. |
l2pRequestId | long | Unique payment request identifier. |
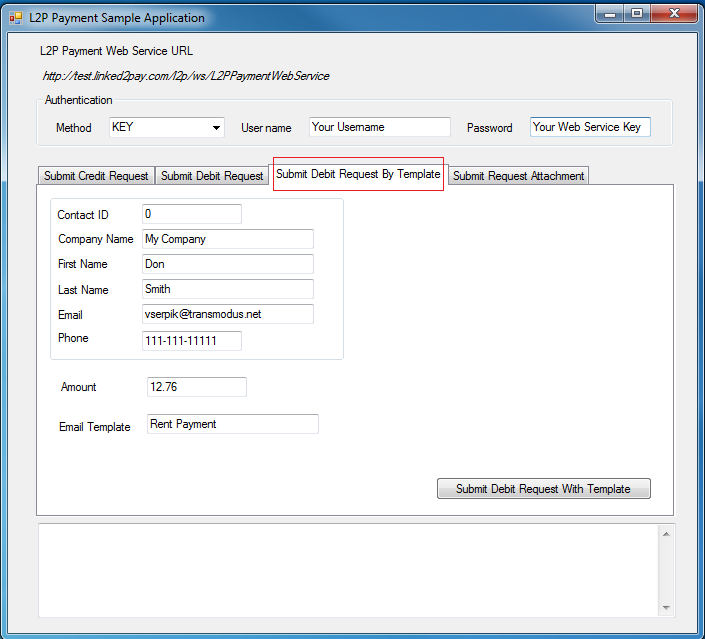
Below is the code sample in PHP
<?php define('L2P_SERVICE_PATH', 'http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT', L2P_SERVICE_PATH.'services/L2PPaymentWebService'); define('L2P_WSDL', L2P_SERVICE_PATH.'wsdl/L2PPaymentWebService.wsdl'); // SOAP client to be used on pages $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object to be used on pages $auth = new StdClass(); $auth->login = "mikel1"; $auth->AuthenticationMethod = "KEY"; $auth->password = "0123456789ABCDEF"; $l2pDebitRequestByTemplate = new StdClass(); $l2pDebitRequestByTemplate->contactId = 0; $l2pDebitRequestByTemplate->companyName = 'My Company'; $l2pDebitRequestByTemplate->firstName = 'Don'; $l2pDebitRequestByTemplate->lastName = 'Smith'; $l2pDebitRequestByTemplate->email = 'vserpik@transmodus.net'; $l2pDebitRequestByTemplate->phone = '111-111-11111'; $l2pDebitRequestByTemplate->amount = 8.50; $l2pDebitRequestByTemplate->emailTemplate = 'Rent Payment'; $params = new StdClass(); $params->auth = $auth; $params->request = $l2pDebitRequestByTemplate; $result = $client->submitDebitRequestByTemplate($params); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Credit Request</title> </head> <body> <div> <p>Response:</p> <div> <?php print_results($result->submitDebitRequestByTemplateReturn); ?> </div> </div> </body> </html> <?php // function to print results function print_results($obj) { foreach ($obj as $k => $v) { print $k.' -- <b>'.$v.'</b><br />'."\n"; } } ?>
Below is the sample code in C#
// Web Reference has been added as: // reference name: L2PReportPaymentWebService // URL: http://test.transmodus.net/jcx/soa/test/wsdl/L2PPaymentWebService.wsdl // More on adding service references see: // http://msdn.microsoft.com/en-us/library/bb628649.aspx // http://msdn.microsoft.com/en-us/library/bb628652.aspx using System; using L2PPaymentSampleApplication.L2PPaymentWebService; namespace L2PPaymentSampleApplication { class Program { static void Main(string[] args) { L2PPaymentWebServiceService l2pPaymentWebService = new L2PPaymentWebServiceService(); ; L2PPaymentWebService.L2PAuthenticationData auth = new L2PPaymentWebService.L2PAuthenticationData(); l2pPaymentWebService.Url = "http://www.linked2pay.com/l2p/ws/L2PPaymentWebService"; L2PPaymentWebServiceService l2pPaymentWebService = new L2PPaymentWebServiceService(); auth.login = "mikel1"; // auth.AuthenticationMethod = "KEY"; // password = "0123456789ABCDEF"; L2PReportWebService.fingerprintBlock fingerprintBlock = new L2PReportWebService.fingerprintBlock(); FingerprintGenerator fingerprintGenerator = new FingerprintGenerator(); fingerprintBlock.sequence = fingerprintGenerator.GetSuquenceNumber(); fingerprintBlock.fingerprint = fingerprintGenerator.GenerateFingerPrint( (int)fingerprintBlock.sequence, fingerprintBlock.timesatmp.ToString(), "0123456789ABCDEF", auth.login); auth.fingerprint = fingerprintBlock; l2pDebitRequestByTemplate.contactId = 0; l2pDebitRequestByTemplate.companyName = "My Company"; l2pDebitRequestByTemplate.firstName = "Don"; l2pDebitRequestByTemplate.lastName = "Smith"; l2pDebitRequestByTemplate.email = "vserpik@transmodus.net";; l2pDebitRequestByTemplate.phone = "111-111-11111"; l2pDebitRequestByTemplate.amount = 8.50; l2pDebitRequestByTemplate.emailTemplate = "Rent Payment"; L2PPaymentWebService.L2PDebitResponse l2pDebitResponse = l2pPaymentWebService.submitDebitRequestByTemplate(auth, l2pDebitRequestByTemplate); Console.WriteLine("Error code: " + l2pDebitResponse.errorCode); Console.WriteLine("Contact ID: " + l2pDebitResponse.l2pContactId); Console.WriteLine("Request ID: " + l2pDebitResponse.l2pRequestId); } } }
The ‘Submit Request Attachment’ method allows you to attach a files or documents to any linked2pay request(s) through an upload process. The attachment file is added to the payment page of the unique request ID that you specify through the linked2pay Webservice.
When your Contact(s) reaches the payment page they will see a link to the file you uploaded. To view the attached file your Contact(s) will simply click on the attachment link, enter the password (if you applied one through the Webservice), and view the file.
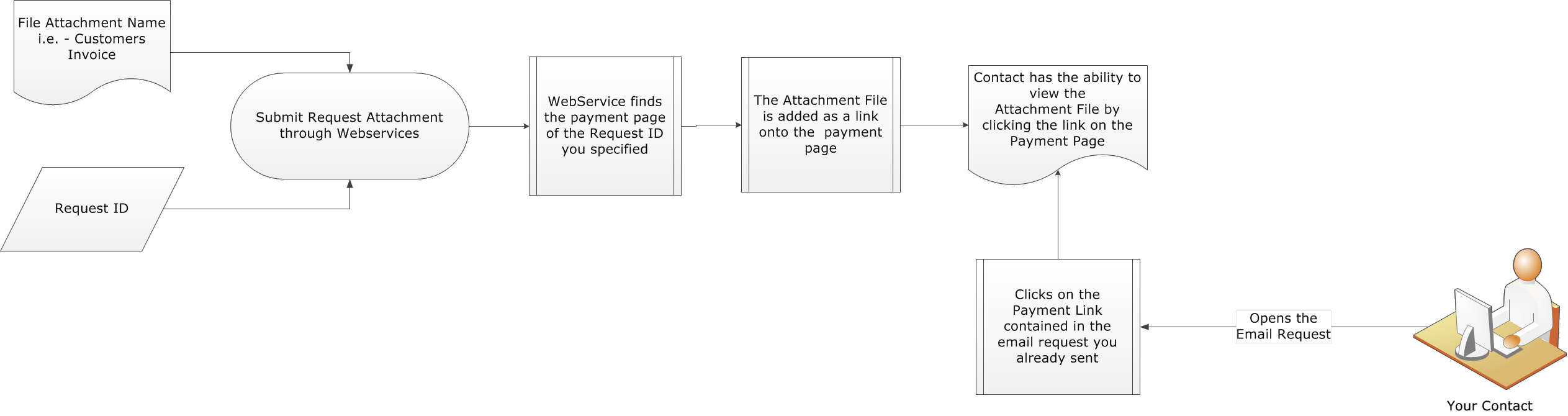
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
attachment | L2PRequestAttachment | Input | Data object that contains attachment attributes. |
attachmentData | base64Binary | Input | Base 64 encoded binary data (type byte[]). |
Returns
Name | Type | Description |
---|---|---|
errorCode | int |
Negative error code or 0 if call is successful. See error codes list in the attachment. |
errorMsg | string | Short explanation of the error code. |
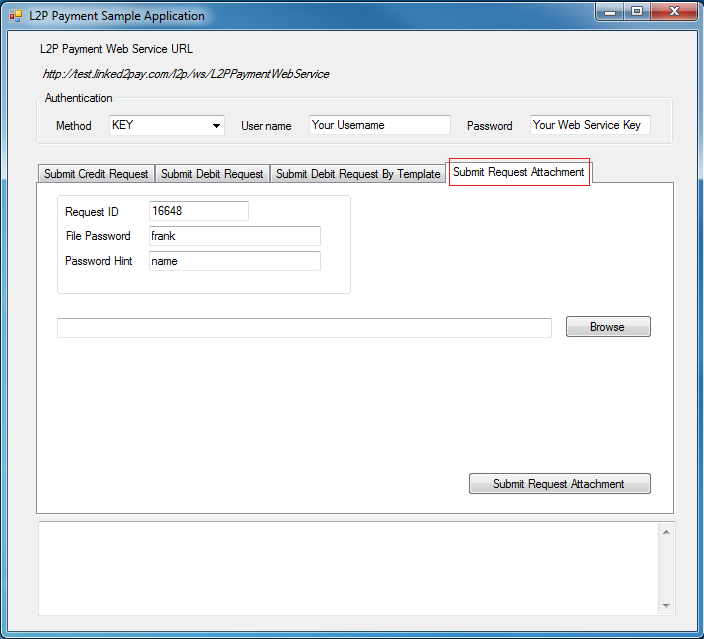
Attachments can be uploaded for both credit and debit requests.
Below is the code sample in PHP
<?php if(isset($_POST['submit'])) { define('L2P_SERVICE_PATH', 'http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT', L2P_SERVICE_PATH.'services/L2PPaymentWebService'); define('L2P_WSDL', L2P_SERVICE_PATH.'wsdl/L2PPaymentWebService.wsdl'); // SOAP client to be used on pages $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object to be used on pages $auth = new StdClass(); $auth->login = "mikel1"; $auth->AuthenticationMethod = "KEY"; $auth->password = "0123456789ABCDEF"; $attachment = new StdClass(); $attachment->fileName = $_FILES['userUpload']['name']; $attachment->filePassword = 'stephen'; $attachment->passwordHint = 'name'; $attachment->requestId = isset($_POST['requestId']) ? $_POST['requestId'] : 1648; $attachmentData = file_get_contents($_FILES['userUpload']['tmp_name']); $encodedData = base64_encode($attachmentData); // These parameters are specific to what function is being called $params = new StdClass(); $params->auth = $auth; $params->attachment = $attachment; $params->attachmentData = $encodedData; $result = $client->submitRequestAttachment($params); } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Submit Request Attachment</title> </head> <body> <div style="background: white;"> <?php if(isset($_POST['submit'])) { ?> <p>Response:</p> <div> <?php print $result->submitRequestAttachmentReturn; ?> </div> <div> </div> <?php } else { ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post" enctype="multipart/form-data"> <div> <input type="file" name="userUpload" /> </div> <div> <input type="submit" name="submit" value="Upload File" /> </div> </form> <?php } ?> </div> </body> </html>
Below is the sample code in C#
// Web Reference has been added as: // reference name: L2PReportPaymentWebService // URL: http://test.transmodus.net/jcx/soa/test/wsdl/L2PPaymentWebService.wsdl // More on adding service references see: // http://msdn.microsoft.com/en-us/library/bb628649.aspx // http://msdn.microsoft.com/en-us/library/bb628652.aspx using System; using L2PPaymentSampleApplication.L2PPaymentWebService; namespace L2PPaymentSampleApplication { class Program { static void Main(string[] args) { L2PPaymentWebServiceService l2pPaymentWebService = new L2PPaymentWebServiceService(); ; L2PPaymentWebService.L2PAuthenticationData auth = new L2PPaymentWebService.L2PAuthenticationData(); L2PPaymentWebServiceService l2pPaymentWebService = new L2PPaymentWebServiceService(); l2pPaymentWebService.Url = "http://www.linked2pay.com/l2p/ws/L2PPaymentWebService"; auth.login = "mikel1"; // auth.AuthenticationMethod = "KEY"; // password = "0123456789ABCDEF"; L2PReportWebService.fingerprintBlock fingerprintBlock = new L2PReportWebService.fingerprintBlock(); FingerprintGenerator fingerprintGenerator = new FingerprintGenerator(); fingerprintBlock.sequence = fingerprintGenerator.GetSuquenceNumber(); fingerprintBlock.fingerprint = fingerprintGenerator.GenerateFingerPrint( (int)fingerprintBlock.sequence, fingerprintBlock.timesatmp.ToString(), "0123456789ABCDEF", auth.login); auth.fingerprint = fingerprintBlock; L2PPaymentWebService.L2PRequestAttachment l2pRequestAttachment = new L2PPaymentWebService.L2PRequestAttachment(); l2pRequestAttachment.requestId = 16648; l2pRequestAttachment.fileName = "test.pdf"; l2pRequestAttachment.filePassword = "stephen"; ll2pRequestAttachment.passwordHint = "name"; // Read attachment file and base-64 encode it. FileStream fs = null; BinaryReader br = null; fs = new FileStream(l2pRequestAttachment.fileName, FileMode.Open, FileAccess.Read); br = new BinaryReader(fs); byte[] bArray = new byte[br.BaseStream.Length]; bArray = br.ReadBytes(Convert.ToInt32(br.BaseStream.Length)); br.Close(); String s64 = Convert.ToBase64String(bArray); byte[] attachmentData = System.Text.Encoding.UTF8.GetBytes(s64); L2PPaymentWebService.L2PAttachmentResponse l2pAttachmentResponse = l2pPaymentWebService.submitRequestAttachment(auth, l2pRequestAttachment, attachmentData); Console.WriteLine("Error code: " + l2pAttachmentResponse .errorCode); } } }
Complex Types
Name | Description |
---|---|
L2PAuthenticationData | Data object that contains authentication information based on user's choice of authentication method. |
L2PCreditRequest | Data object that defines payment request. |
L2PCreditResponse | Response object with information on submitted request. |
L2PDebitRequest | Data object that defines payment request. |
L2PDebitRequestByTemplate | Data object that defines payment request. |
L2PDebitResponse | Response object with information on submitted request. |
L2PRequestAttachment | Data object that contains attachment attributes. |
L2PAttachmentResponse | Response object with information on submitted request. |
ReqFieldsType | Array of variables that consist of ReqFieldType |
ReqFieldType | Represents custom variables and values associated with a linked2pay payment request template |
fingerprintBlock | A bundle of information, containing user's sequence number, timestamp and a 16-character generated fingerprint |
Description
Data object that defines payment request.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
contactId | long | 1..1 | This parameter can be specified if caller knows L2P system ID of the existing contact. Otherwise it has to be set to 0 and system determines contact by email address. |
companyName | string | 1..1 |
Contacts company name. Maximum length is 50 characters. This field is optional. |
firstName | string | 1..1 |
Contact first name. Maximum length is 50 characters. This field is required. |
lastName | string | 1..1 |
Contact last name. Maximum length is 50 characters. This field is required. |
string | 1..1 |
Contact email address. System checks if contact with this email address already exists and updates his attributes (first/last names, company name, cc/bcc addresses and phone). If contact with this email address does not exist then the new contact is created. Email address is validated to match common email address syntax. Maximum length of email address is 50 characters. This field is required. |
|
cc | string | 1..1 |
Maximum length is 50 characters. This field is optional. |
bcc | string | 1..1 |
Maximum length is 50 characters. This field is optional. |
phone | string | 1..1 |
Maximum length is 20 characters. This field is optional. |
amount | double | 1..1 | This field is required. |
emailMessage | string | 1..1 |
Custom message that is included in standard notification email sent to the contact(s). Maximum length is 15000 characters. This field is optional. |
address1 | string | 1..1 |
Maximum length is 10 characters. This field is required. |
address2 | string | 1..1 |
Maximum length is 10 characters. This field is optional. |
city | string | 1..1 |
Maximum length is 10 characters. This field is required. |
state | string | 1..1 |
Maximum length is 2 characters. This field is required. |
zip | string | 1..1 |
Maximum length is 10 characters. Zip code is validated to match common zip code syntax. This field is required. |
invoices | string | 1..1 |
Maximum length is 50 characters. This field is optional. |
glBank | string | 1..1 |
Maximum length is 50 characters. This field is optional. |
glExpense | string | 1..1 |
Maximum length is 50 characters. This field is optional. |
glDesc | string | 1..1 |
Maximum length is 14000 characters. This field is optional. |
Description
Response object with information on submitted request.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
errorCode | int | 1..1 |
Negative error code or 0 if call is successful. See error codes list in the attachment. |
errorMsg | string | 1..1 | Short explanation of the error code. |
l2pContactId | long | 1..1 | Unique contact identifier |
l2pRequestId | long | 1..1 | Unique payment request identifier |
Description
Data object that defines payment request.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
contactId | long | 1..1 | This parameter can be specified if caller knows L2P system ID of the existing contact. Otherwise it has to be set to 0 and system determines contact by email address. |
companyName | string | 1..1 |
Contacts' company name. Maximum length is 50 characters. This field is optional. |
firstName | string | 1..1 |
Contact first name. Maximum length is 50 characters. This field is required. |
lastName | string | 1..1 |
Contact last name. Maximum length is 50 characters. This field is required. |
string | 1..1 |
Contact email address. System checks if contact with this email address already exists and updates his attributes (first/last names, company name, cc/bcc addresses and phone). If contact with this email address does not exist then the new contact is created. Email address is validated to match common email address syntax. Maximum length of email address is 50 characters. This field is required. |
|
phone | string | 1..1 |
Maximum length is 20 characters. This field is optional. |
amount | double | 1..1 | This field is required. |
emailSubject | string | 1..1 |
Maximum length is 100 characters. This field is required. |
emailBody | string | 1..1 |
Maximum length is 1050 characters. This field is required. |
Description
Data object that defines payment request.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
contactId | long | 1..1 | This parameter can be specified if caller knows L2P system ID of the existing contact. Otherwise it has to be set to 0 and system determines contact by email address. |
companyName | string | 1..1 |
Contacts' company name. Maximum length is 50 characters. This field is optional. |
firstName | string | 1..1 |
Contact first name. Maximum length is 50 characters. This field is required. |
lastName | string | 1..1 |
Contact last name. Maximum length is 50 characters. This field is required. |
string | 1..1 |
Contact email address. System checks if contact with this email address already exists and updates his attributes (first/last names, company name, cc/bcc addresses and phone). If contact with this email address does not exist then the new contact is created. Email address is validated to match common email address syntax. Maximum length of email address is 50 characters. This field is required. |
|
phone | string | 1..1 |
Maximum length is 20 characters. This field is optional. |
amount | double | 1..1 | This field is required. |
emailTemplate | string | 1..1 |
Name of the existing email template. Maximum length is 50 characters. This field is required. |
templateVariables | Map | 1..1 |
Email template can include variables (placeholders). For example "This payment is for invoice [invoice]" that should be sent as "This payment is for invoice 123". When calling web service caller should list all template variables and their values. Parameter templateVariables is a HashMap - collection of items. Each item represents a pair of template variable name and its value. |
Description
Response object with information on submitted request.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
errorCode | int | 1..1 |
Negative error code or 0 if call is successful. See error codes list in the attachment. |
errorMsg | string | 1..1 | Short explanation of the error code. |
l2pContactId | long | 1..1 | Unique contact identifier |
l2pRequestId | long | 1..1 | Unique payment request identifier |
Description
Data object that contains attachment attributes.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
requestId | long | 1..1 |
Request ID of a payment request that the submitted attachment will be associated with. This field is required. |
filePassword | string | 1..1 |
Password up to 20 characters. If specified it will be requested on the L2P payment page to open/view the attachment. This field is optional. |
passwordHint | long | 1..1 |
Password hint up to 50 characters. If specified it will be displayed on the L2P payment page above the entry field for the file password. This field is optional. |
fileName | long | 1..1 |
Name of the attachment file. System uses file name extension to detect attachment type (pdf, txt, etc.) This field is required. |
Description
Response object with information on the submitted request.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
errorCode | int | 1..1 |
Negative error code or 0 if call is successful. See error codes list in the attachment. |
errorMsg | string | 1..1 | Short explanation of the error code. |
Description
Array of variables that consist of ReqFieldType.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
Field | ReqFieldType | 1..* | List of request payment fields. |
Description
Represents custom variables and values associated with a linked2pay payment request template.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
Label | string | 1..1 | Label associated with the template field. |
Value | string | 1..1 | Value associated with the template field. |
Other Elements
Web Service Possible Error Codes
This is a list of possible error codes you may see when using the Online Forms, Email Payments, or Reporting Web Services.
ERROR CODES
Description
Negative error code or 0 if call is successful.
See full list of possible error codes list below:
Note: This list of error codes applies to the L2P Widget Webservice, L2P Payment Webservice, and the L2P Reporting Webservice
Possible error codes:
L2P_OK = 0
L2P_DB_EXCP = -1
L2P_USER_NOT_EXIST = -2
L2P_USER_ACCESS_DENIED = -3
L2P_REQUEST_NOT_EXIST = -4
L2P_REQUEST_ACCESS_DENIED = -5
L2P_NO_REQUESTS_FOUND = -6
L2P_CONTACT_NOT_EXIST = -7
L2P_EMAIL_FAILED = -8
L2P_CONTACT_OPT_OUT = -9
L2P_EMAIL_TOO_LONG = -10
L2P_PAYMENT_URL_MISSING = -11
L2P_SYSTEM_ERROR = -12
L2P_HEADER_NOT_EXIST = -13
L2P_NO_ATTACHMENT = -14
L2P_NO_HEADERS_FOUND = -15
L2P_NO_WIDGETS_FOUND = -16
L2P_COMPANY_NAME_TOO_LONG = -17
L2P_LAST_NAME_NOT_PRESENT = -18
L2P_LAST_NAME_TOO_LONG = -19
L2P_FIRST_NAME_NOT_PRESENT = -20
L2P_FIRST_NAME_TOO_LONG = -21
L2P_EMAIL_INVALID = -22
L2P_EMAIL_NOT_PRESENT = -23
L2P_AMOUNT_NOT_PRESENT = -24
L2P_CC_INVALID = -25
L2P_CC_TOO_LONG = -26
L2P_BCC_INVALID = -27
L2P_BCC_TOO_LONG = -28
L2P_PHONE_TOO_LONG = -29
L2P_TEMPLATE_NAME_TOO_LONG = -30
L2P_EMAIL_SUBJECT_TOO_LONG = -31
L2P_EMAIL_BODY_TOO_LONG = -32
L2P_ADDRESS1_NOT_PRESENT = -33
L2P_ADDRESS1_TOO_LONG = -34
L2P_ADDRESS2_TOO_LONG = -35
L2P_CITY_NOT_PRESENT = -36
L2P_CITY_TOO_LONG = -37
L2P_STATE_NOT_PRESENT = -38
L2P_STATE_TOO_LONG = -39
L2P_ZIP_NOT_PRESENT = -40
L2P_ZIP_TOO_LONG = -41
L2P_ZIP_INVALID = -42
L2P_GL_BANK_TOO_LONG = -43
L2P_GL_EXPENSE_TOO_LONG = -44
L2P_GL_DESC_TOO_LONG = -45
L2P_INVOICE_TOO_LONG = -46
L2P_EMAIL_MESSAGE_TOO_LONG = -47
L2P_TEMPLATE_INVALID = -48
L2P_EMAIL_SUBJECT_NOT_PRESENT = -49
L2P_EMAIL_BODY_NOT_PRESENT = -50
L2P_WIDGET_REQUEST_INVALID_HEADER = -101
L2P_WIDGET_REQUEST_INVALID_LOOKUP1 = -102
L2P_WIDGET_REQUEST_INVALID_LOOKUP2 = -103
L2P_WIDGET_REQUEST_INVALID_DESCRIPTION = -104
L2P_WIDGET_REQUEST_INVALID_START_DATE = -105
L2P_WIDGET_REQUEST_INVALID_END_DATE = -106
L2P_WIDGET_REQUEST_INVALID_PAST_DUE_DATE = -107
L2P_WIDGET_REQUEST_INVALID_AMOUNT = -108
L2P_WIDGET_REQUEST_INVALID_PASTDUE_AMOUNT = -109
L2P_WIDGET_REQUEST_INVALID_PHONE_NUMBER = -110
L2P_WIDGET_REQUEST_INVALID_PYMT_LINK = -111
L2P_WIDGET_REQUEST_INVALID_PHONE_NUMBER_OR_PYMT_LINK = -112
L2P_WIDGET_REQUEST_INVALID_EMAIL = -113
L2P_WIDGET_REQUEST_INVALID_OPTOUT_EMAIL = -114
L2P_WIDGET_REQUEST_INVALID_STREET1 = -115
L2P_WIDGET_REQUEST_INVALID_STREET2 = -116
L2P_WIDGET_REQUEST_INVALID_CITY = -117
L2P_WIDGET_REQUEST_INVALID_STATE = -118
L2P_WIDGET_REQUEST_INVALID_ZIP = -119
L2P_WIDGET_REQUEST_INVALID_ADDRESS = -120
L2P_WIDGET_REQUEST_INVALID_USER = -121
L2P_WIDGET_REQUEST_INVALID_USER_ACCOCIATION = -122
L2P_WIDGET_REQUEST_INVALID_WIDGET = -123
L2P_WIDGET_REQUEST_INVALID_MOBILE_NOLOOKUP_NOMAP_TEMPLATE = -124
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_LOOKUP1_REQUIRED = -125
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_ADDRESS1_REQUIRED = -126
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_CITY_REQUIRED = -127
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_STATE_REQUIRED = -128
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_ZIP_REQUIRED = -129
L2P_ATTACHMENT_INVALID_PASSWORD = -140
L2P_ATTACHMENT_INVALID_HINT = -141
L2P_ATTACHMENT_INVALID_FILE_NAME = -142
L2P_WIDGET_DATA_ID_NOT_PRESENT = -143
L2P_REQUEST_ID_NOT_PRESENT = -144
Send Credit Requests
Use the Email Payments WebService to easily send a payment to someone via email. The recipient accepts the credit to their account on our secure payment page, you and your payee receive a payment receipt via email and the loop is closed.
Send Debit Requests
Quickly submit a debit request by calling our Email Payments WebService. linked2pay will deliver a debit request to the email you specified with a link to our secure payment page directing payment to your company.
Resource Quick Links